We’re serving to a shopper that has a WordPress WooCommerce web site that was shedding search engine visibility for years as a result of a whole bunch of code, configuration, and content material points from years of neglect, put in and uninstalled plugins, and dozens of themes.
With the brand new web site launched, we’ve been observing the efficiency of the location and just lately acquired the next Google Search Console message:
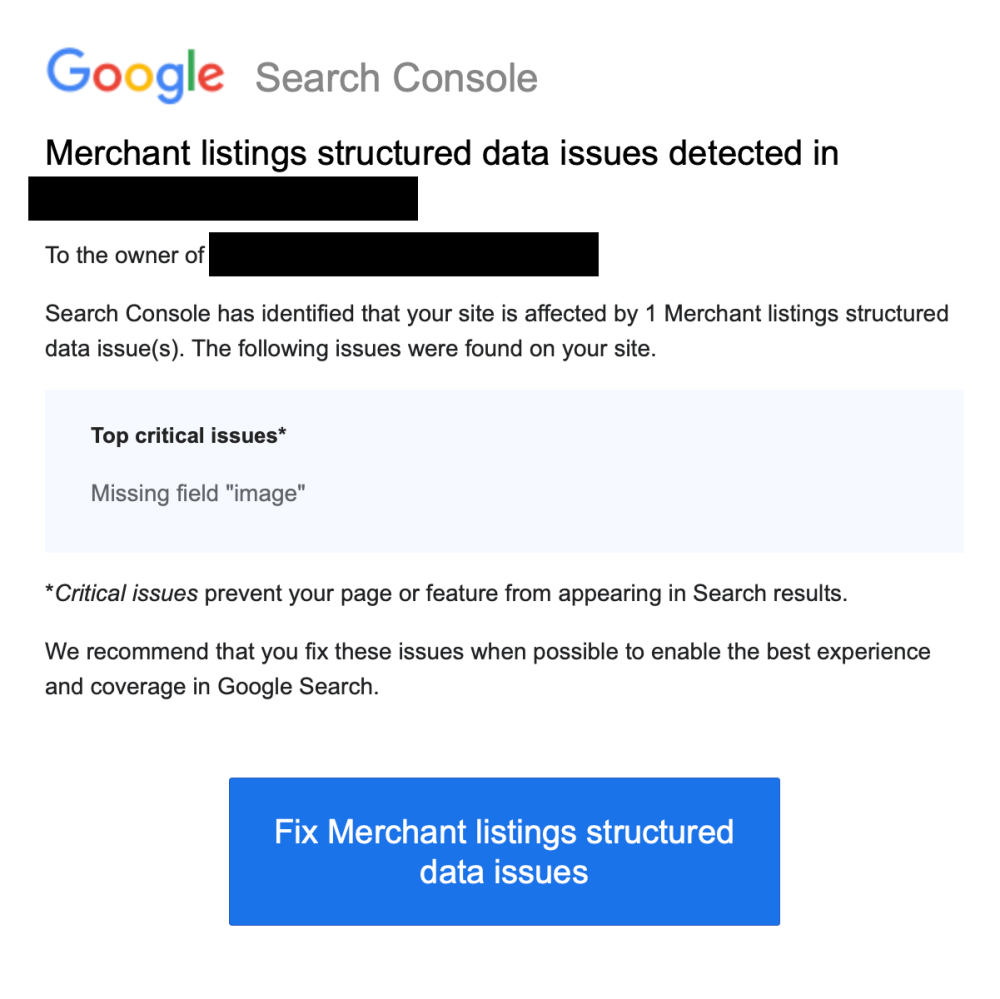
We had been stunned that the corporate had merchandise listed in WooCommerce that didn’t have a product picture set. Once we crawled the newly launched web site, we didn’t see any issues… this was as a result of the brand new theme had a placeholder picture that appeared each time a picture was not set. Consequently, there have been no errors for photos not discovered.
WooCommerce Merchandise Listing
Our subsequent step was to determine the merchandise throughout the web site the place there have been no photos set. That’s not a straightforward activity when there are a whole bunch of merchandise to filter via. Consequently, we wrote our personal filter in WooCommerce merchandise to filter the record to merchandise with no product picture set.
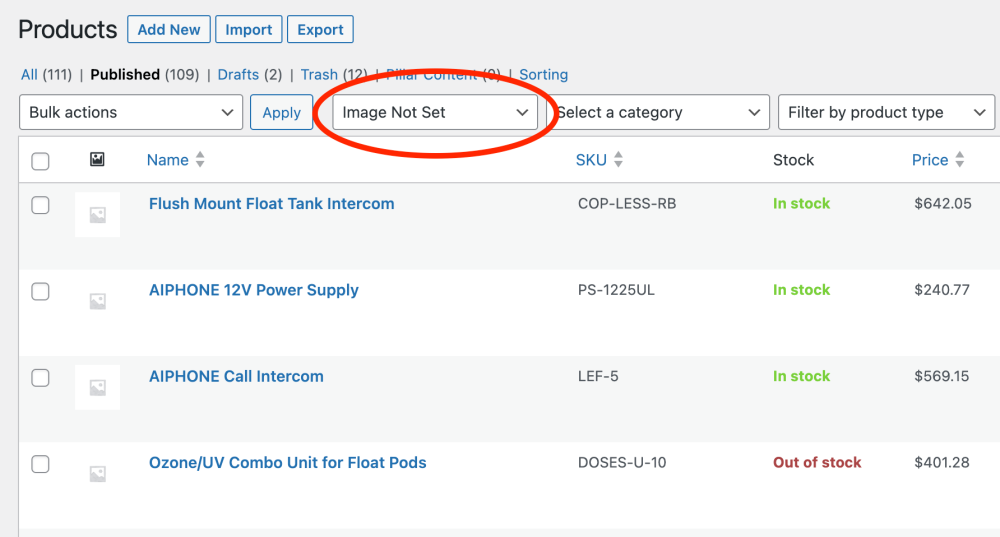
Now we are able to simply browse the record and replace the product photos the place essential with out effort. Right here’s how we did it.
Add Filter To WooCommerce Admin Merchandise Listing
Throughout the shopper’s youngster theme capabilities.php web page, we added the next two sections of code. First, we construct the filter discipline:
// Add a filter on product for set product picture
add_action('restrict_manage_posts', 'filter_products_by_image_presence');
perform filter_products_by_image_presence() {
world $typenow;
$chosen = isset($_GET['product_image_presence']) ? $_GET['product_image_presence'] : '';
if ('product' === $typenow) {
?>
<choose identify="product_image_presence" id="product_image_presence">
<possibility worth="">Filter by product picture</possibility>
<possibility worth="set" <?php chosen('set', $chosen); ?>>Picture Set</possibility>
<possibility worth="notset" <?php chosen('notset', $chosen); ?>>Picture Not Set</possibility>
</choose>
<?php
}
}
Right here’s a step-by-step clarification of what every a part of the code does:
add_action('restrict_manage_posts', 'filter_products_by_image_presence');
- This line hooks into
restrict_manage_posts
, which is an motion triggered within the WordPress admin space, permitting you so as to add further filtering choices to the posts record. Right here, it’s used so as to add a brand new filter to the WooCommerce merchandise record.
- This line hooks into
perform filter_products_by_image_presence() { ... }
- This block defines the perform
filter_products_by_image_presence
, which outputs HTML for a brand new dropdown choose filter on the product admin display screen.
- This block defines the perform
world $typenow;
- The worldwide variable
$typenow
is used to examine the kind of the present submit record to make sure that the customized filter is simply added to the ‘Merchandise’ submit sort screens and never others.
- The worldwide variable
$chosen = isset($_GET['product_image_presence']) ? $_GET['product_image_presence'] : '';
- This line checks if there may be an energetic filter set by in search of the ‘product_image_presence’ parameter within the URL, which is handed as a GET request when you choose a filter possibility and submit the filter. It shops the present choice to retain the chosen state of the filter after the web page reloads.
if ('product' === $typenow) { ... }
- This conditional assertion checks whether or not the present submit sort is ‘product’, making certain the code contained in the if-statement solely runs for WooCommerce merchandise.
- Every part between
?>
and<?php
is HTML output, together with the choose dropdown with choices for filtering by merchandise with ‘Picture Set’ or ‘Picture Not Set’. PHP is interspersed to deal with dynamic choice by way of thechosen()
perform, which outputs thechosen
attribute if the present$chosen
worth matches the choice worth. - The
chosen()
perform is a WordPress helper perform that compares the primary argument with the second and in the event that they match, it outputs ‘chosen=”chosen”‘, which is the HTML attribute wanted to point out an possibility as chosen in a dropdown.
This code successfully provides a dropdown filter to the merchandise record, enabling the admin to filter the record by merchandise which have a picture set or not. This extra filter would assist customers handle massive catalogs, making certain merchandise adhere to retailer itemizing necessities, together with having photos assigned as a part of the itemizing high quality management.
Execute Question on WooCommerce Admin Merchandise Listing
Subsequent, we now have so as to add a question that may execute and discover the merchandise that haven’t any picture set.
add_filter('parse_query', 'filter_products_query_by_image_presence');
perform filter_products_query_by_image_presence($question) {
world $pagenow, $typenow;
if ('edit.php' === $pagenow && 'product' === $typenow && isset($_GET['product_image_presence']) && $_GET['product_image_presence'] != '') {
$presence = $_GET['product_image_presence'];
$meta_query = array(
'relation' => 'OR',
array(
'key' => '_thumbnail_id',
'examine' => 'NOT EXISTS'
),
array(
'key' => '_thumbnail_id',
'worth' => '0'
)
);
if ('set' === $presence) {
$meta_query = array(
array(
'key' => '_thumbnail_id',
'examine' => 'EXISTS'
),
array(
'key' => '_thumbnail_id',
'worth' => array('', '0'), // Assuming '0' or '' could possibly be placeholders for no picture.
'examine' => 'NOT IN'
),
);
} elseif ('notset' === $presence) {
$meta_query = array(
'relation' => 'OR',
array(
'key' => '_thumbnail_id',
'examine' => 'NOT EXISTS'
),
array(
'key' => '_thumbnail_id',
'worth' => '0'
)
);
}
$query->set('meta_query', $meta_query);
}
}
This code snippet is for modifying the principle WordPress question for product listings within the admin space to permit filtering merchandise based mostly on whether or not they have an related picture. Right here’s a proof of its elements:
add_filter('parse_query', 'filter_products_query_by_image_presence');
- This line attaches the
filter_products_query_by_image_presence
perform to theparse_query
filter hook, which is used to regulate the principle question that WordPress makes use of to retrieve posts (or customized submit sorts like merchandise) within the admin record desk.
- This line attaches the
perform filter_products_query_by_image_presence($question) { ... }
- This perform is outlined to change the product record question based mostly on the presence of product photos. The
$question
variable is an occasion of theWP_Query
class, handed by reference, which implies any adjustments to this object will have an effect on the precise question WordPress runs.
- This perform is outlined to change the product record question based mostly on the presence of product photos. The
world $pagenow, $typenow;
- These world variables are WordPress setting variables.
$pagenow
is used to examine the present admin web page, and$typenow
to examine the present submit sort. - The conditional assertion checks if the present web page is ‘edit.php’ (the default web page for itemizing posts and customized submit sorts), the submit sort is ‘product’ (which implies we’re on the WooCommerce merchandise record), and if a filter has been set via a
GET
parameter named ‘product_image_presence’.
- These world variables are WordPress setting variables.
- A brand new meta question array is created based mostly on the worth of ‘product_image_presence’. This array is designed to create the circumstances for filtering merchandise with or with out photos.
- The
relation
key set to ‘OR’ signifies that any of the circumstances inside might be true for the meta question to retrieve the merchandise. - If the filter is about to ‘set’, a brand new
$meta_query
is created to search out merchandise with photos. Merchandise which have a ‘_thumbnail_id’ (which implies a picture is about) and never an empty string or ‘0’ are included. - If the filter is about to ‘notset’, the meta question seems for merchandise the place the ‘_thumbnail_id’ meta key both doesn’t exist or is about to ‘0’, which might imply there isn’t a picture related to the product.
- The
$query->set('meta_query', $meta_query);
- This line modifies the principle question by setting the ‘meta_query’ with the circumstances outlined in
$meta_query
.
- This line modifies the principle question by setting the ‘meta_query’ with the circumstances outlined in
This customization helps a WooCommerce retailer admin to shortly discover merchandise missing photos, which is essential for stock administration, advertising and marketing, and gross sales methods, as merchandise with photos usually tend to promote and supply prospects with the mandatory visible info. By making certain product listings are full with photos, gross sales and advertising and marketing efforts might be more practical.