A WordPress plugin includes extra overhead, requiring WordPress to load and handle the plugin. This contains studying the plugin listing, checking for updates, and sustaining plugin metadata. Every time I’m engaged on optimizing Martech Zone or a shopper’s WordPress set up, I at all times analyze the plugins utilized, high quality of the code, impression on the positioning, and the way a lot performance there’s within the plugin getting used.
I typically discover that plugins are bloated, poorly developed, and add pointless overhead that may decelerate the positioning or administration. If it’s a easy function, I’ll usually take away the plugin and modify the kid theme capabilities.php as an alternative. When code is positioned within the little one theme’s capabilities.php
file, it’s instantly built-in into the theme, which may be barely extra environment friendly concerning useful resource utilization.
In the end, your selection also needs to contemplate components like code group, upkeep, and your challenge’s particular wants.
Add Featured Picture Column To Posts Listing
I used to be operating a plugin on Martech Zone which added a column to the Posts Listing with the featured picture. Sadly, the plugin had some bloat with pointless extra settings and sources like a video that had lengthy been taken down. I dissected the plugin and found out how they had been including the picture column… after which I modified the plugin with some extra options like having the title and dimensions of the featured picture upon mouseover.
Right here’s the code:
operate add_featured_image_column($columns) {
// Create a brand new column with the identify "img"
$columns['img'] = 'Featured Picture';
return $columns;
}
operate customize_featured_image_column($column_name, $post_id) {
if ($column_name == 'img') {
// Get the featured picture URL
$thumbnail_url = get_the_post_thumbnail_url($post_id, 'thumbnail');
// Test if a featured picture is about
if ($thumbnail_url) {
// Get the unique picture URL
$original_url = get_the_post_thumbnail_url($post_id, 'full');
// Get the scale of the unique picture
checklist($original_width, $original_height) = getimagesize($original_url);
// Get the precise title
$actual_title = get_the_title($post_id);
// Outline the title attribute for the picture
$image_title = $actual_title . ' (' . $original_width . 'px by ' . $original_height . 'px)';
// Show the thumbnail picture with a most peak of 80px and add dimensions to the title attribute
echo '<img src="' . esc_url($thumbnail_url) . '" fashion="max-height: 80px;" title="' . $image_title . '" />';
} else {
// No featured picture is about, show "No featured picture"
echo 'No featured picture';
}
}
return $column_name;
}
add_action('manage_posts_columns', 'add_featured_image_column');
add_action('manage_posts_custom_column', 'customize_featured_image_column', 10, 2);
Right here’s a proof of the code:
add_featured_image_column
operate:- This operate provides a customized column to the checklist of posts within the WordPress admin panel. It takes an array of present columns as an argument (
$columns
). - It provides a brand new column named img with the label Featured Picture.
- It returns the modified array of columns with the brand new img column added.
- This operate provides a customized column to the checklist of posts within the WordPress admin panel. It takes an array of present columns as an argument (
customize_featured_image_column
operate:- This operate is chargeable for customizing the content material of the img column for every publish within the checklist.
- It takes two parameters:
$column_name
(the identify of the present column being processed) and$post_id
(the ID of the present publish). - It checks if the at the moment processed column is img (the customized column we added).
- If it’s the img column, it fetches and shows the featured picture and extra info.
- It makes use of
get_the_post_thumbnail_url
to retrieve the URL of the featured picture within the “thumbnail” measurement. - It checks if a featured picture is about by verifying if the
$thumbnail_url
just isn’t empty. - If a featured picture is about, it additionally retrieves the URL of the unique (full-sized) picture and its dimensions utilizing
get_the_post_thumbnail_url
andgetimagesize
. - It fetches the precise title of the publish utilizing
get_the_title
. - It constructs the
title
attribute for the picture within the format “Title: Precise Title (Authentic Width px by Authentic Peak px).” - It shows the thumbnail picture with a most peak of 80px and units the title attribute to the constructed
$image_title
. You’ll be able to modify this peak to no matter you’d like. - If no featured picture is about, it shows “No featured picture set.”
- The operate returns the modified content material for the img column.
add_action
strains:- These strains hook the
add_featured_image_column
operate to themanage_posts_columns
motion and thecustomize_featured_image_column
operate to themanage_posts_custom_column
motion. This associates these capabilities with the WordPress publish administration display screen.
- These strains hook the
By including these actions, the code successfully creates a brand new customized column within the WordPress admin posts checklist that shows the featured picture of every publish together with its title and dimensions. If there isn’t a featured picture, it shows No featured picture. This is usually a useful function for managing and reviewing posts within the admin space, particularly when working with themes that closely depend on featured photos.
Right here’s a preview of this in motion on Martech Zone the place I’m mousing over the featured picture within the third row:
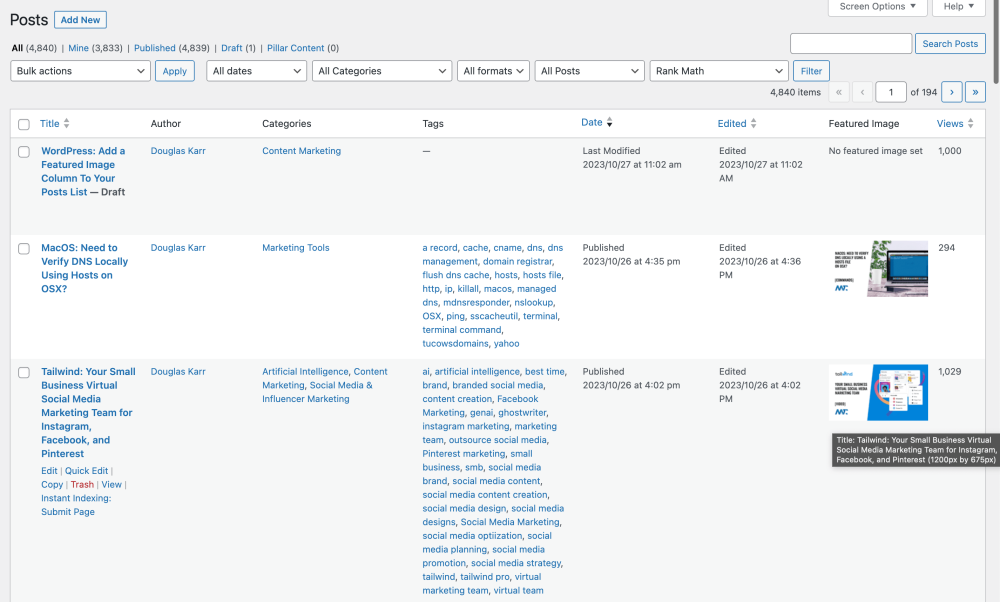
Filter For Posts With No Featured Picture
One other operate that I added was a filter in order that I might simply establish any publish that had no featured picture set.
operate add_no_featured_image_filter() {
international $post_type;
// Test if the present publish sort is 'publish' (you may change it to the specified publish sort)
if ($post_type == 'publish') {
$chosen = (isset($_GET['no_featured_image']) && $_GET['no_featured_image'] == '1') ? 'chosen' : '';
echo '<choose identify="no_featured_image" id="no_featured_image">
<choice worth="" ' . $chosen . '>All Posts</choice>
<choice worth="1" ' . chosen('1', $_GET['no_featured_image'], false) . '>No Featured Picture</choice>
</choose>';
}
}
operate filter_no_featured_image_posts($question) {
international $pagenow;
// Test if we're on the posts web page and the filter is about
if (is_admin() && $pagenow == 'edit.php' && isset($_GET['no_featured_image']) && $_GET['no_featured_image'] == '1') {
$query->set('meta_key', '_thumbnail_id');
$query->set('meta_compare', 'NOT EXISTS');
}
}
add_action('restrict_manage_posts', 'add_no_featured_image_filter');
add_action('parse_query', 'filter_no_featured_image_posts');
This code enhances the performance of the WordPress admin space by including a customized filter for posts to permit customers to filter posts based mostly on whether or not they have a featured picture set or not. Right here’s a proof of the code:
add_no_featured_image_filter
operate:- This operate creates a customized filter dropdown for posts within the WordPress admin panel.
- It begins by checking the present publish sort. On this code, it’s particularly checking if the present publish sort is “publish,” however you may change this to any desired publish sort.
- If the publish sort matches, it proceeds to generate the filter dropdown.
- The filter dropdown is an HTML
<choose>
component with the identify “no_featured_image” and the ID “no_featured_image.” - It comprises two choices:
- “All Posts” (default choice): This selection is chosen when no particular filtering is utilized.
- “No Featured Picture”: This selection is chosen when the person needs to filter posts with no featured picture.
- The collection of these choices is decided based mostly on the URL question parameters (
$_GET
) and whether or not theno_featured_image
parameter is about to ‘1’. - The
chosen
operate is used to find out whether or not an choice needs to be marked as “chosen” based mostly on the question parameter values. - The operate echoes the HTML for the filter dropdown.
filter_no_featured_image_posts
operate:- This operate modifies the question to filter posts based mostly on the presence or absence of a featured picture.
- It first checks if we’re within the WordPress admin space and on the “edit.php” web page, which is the posts administration web page.
- It then checks if the
no_featured_image
question parameter is about to ‘1’, indicating that the person needs to filter posts with no featured picture. - If the filter is lively, it makes use of the
set
methodology to switch the question: - It units the
meta_key
to _thumbnail_id, the important thing used to retailer the featured picture ID within the publish’s metadata. - It units the
meta_compare
to ‘NOT EXISTS,’ which successfully filters posts the place the ‘_thumbnail_id’ meta key doesn’t exist. In different phrases, it filters posts with no featured picture. - This operate adjusts the question based mostly on the filter choice.
add_action
strains:- The primary
add_action
line hooks theadd_no_featured_image_filter
operate to the ‘restrict_manage_posts’ motion. This motion is named when displaying the publish administration part, and it lets you add customized filters and controls. - The second
add_action
line hooks thefilter_no_featured_image_posts
operate to the ‘parse_query’ motion. This motion is named earlier than the question is executed, permitting you to switch the question based mostly on customized filters.
- The primary
Right here’s a preview of the filter:
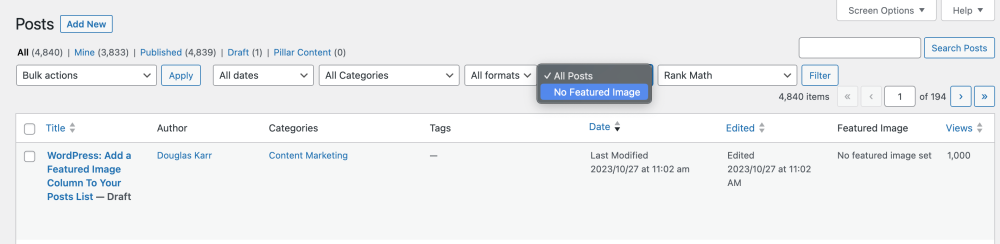
By including these actions, the code creates a customized filter within the WordPress admin posts checklist that lets you filter posts based mostly on whether or not they have a featured picture set or not, making it simpler to handle and set up your posts.